-
-
Notifications
You must be signed in to change notification settings - Fork 18
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Browse files
Browse the repository at this point in the history
Resolves #33 This PR changes the fetching algorithm significantly in an attempt to improve the final output. Specifically, 1. Information about assets of all tools is fetched first before downloading the tools. This way users see all validation errors before the downloading has started. > The only validation errors left are missing `exe` files in assets, I believe. Or GitHub Rate-Limit error when trying to download an asset. 2. Estimated download size is printed before the downloading (so users can see how much it would take to download). 3. The correct tags are shown immediately. 4. Lots of refactoring. A single GIF worth a thousand words: 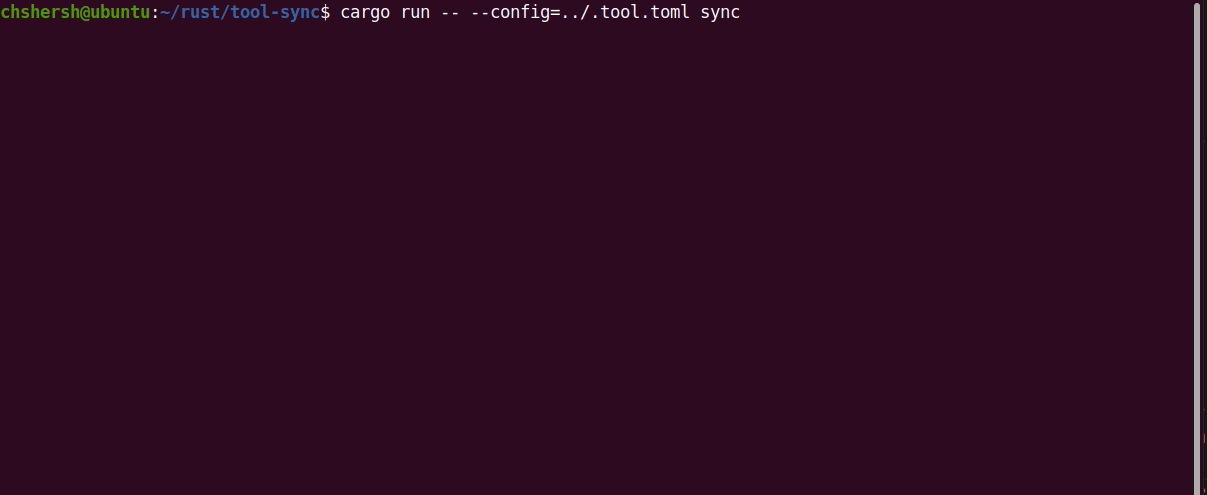
- Loading branch information
Showing
11 changed files
with
471 additions
and
242 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1 @@ | ||
pub mod client; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,103 @@ | ||
use std::env; | ||
use std::error::Error; | ||
use std::io::Read; | ||
|
||
use crate::model::release::{Asset, Release}; | ||
|
||
/// GitHub API client to handle all API requests | ||
pub struct Client { | ||
pub owner: String, | ||
pub repo: String, | ||
pub version: String, | ||
} | ||
|
||
impl Client { | ||
fn release_url(&self) -> String { | ||
format!( | ||
"https://api.github.com/repos/{owner}/{repo}/releases/{version}", | ||
owner = self.owner, | ||
repo = self.repo, | ||
version = self.version, | ||
) | ||
} | ||
|
||
fn asset_url(&self, asset_id: u32) -> String { | ||
format!( | ||
"https://api.github.com/repos/{owner}/{repo}/releases/assets/{asset_id}", | ||
owner = self.owner, | ||
repo = self.repo, | ||
asset_id = asset_id | ||
) | ||
} | ||
|
||
pub fn fetch_release_info(&self) -> Result<Release, Box<dyn Error>> { | ||
let release_url = self.release_url(); | ||
|
||
let req = add_auth_header( | ||
ureq::get(&release_url) | ||
.set("Accept", "application/vnd.github+json") | ||
.set("User-Agent", "chshersh/tool-sync-0.2.0"), | ||
); | ||
|
||
let release: Release = req.call()?.into_json()?; | ||
|
||
Ok(release) | ||
} | ||
|
||
pub fn get_asset_stream( | ||
&self, | ||
asset: &Asset, | ||
) -> Result<Box<dyn Read + Send + Sync>, ureq::Error> { | ||
let asset_url = self.asset_url(asset.id); | ||
|
||
let req = add_auth_header( | ||
ureq::get(&asset_url) | ||
.set("Accept", "application/octet-stream") | ||
.set("User-Agent", "chshersh/tool-sync-0.2.0"), | ||
); | ||
|
||
Ok(req.call()?.into_reader()) | ||
} | ||
} | ||
|
||
fn add_auth_header(req: ureq::Request) -> ureq::Request { | ||
match env::var("GITHUB_TOKEN") { | ||
Err(_) => req, | ||
Ok(token) => req.set("Authorization", &format!("token {}", token)), | ||
} | ||
} | ||
|
||
#[cfg(test)] | ||
mod tests { | ||
use super::*; | ||
|
||
use crate::model::tool::ToolInfoTag; | ||
|
||
#[test] | ||
fn release_url_with_latest_tag_is_correct() { | ||
let client = Client { | ||
owner: String::from("OWNER"), | ||
repo: String::from("REPO"), | ||
version: ToolInfoTag::Latest.to_str_version(), | ||
}; | ||
|
||
assert_eq!( | ||
client.release_url(), | ||
"https://api.github.com/repos/OWNER/REPO/releases/latest" | ||
); | ||
} | ||
|
||
#[test] | ||
fn release_url_with_specific_tag_is_correct() { | ||
let client = Client { | ||
owner: String::from("OWNER"), | ||
repo: String::from("REPO"), | ||
version: ToolInfoTag::Specific(String::from("SPECIFIC_TAG")).to_str_version(), | ||
}; | ||
|
||
assert_eq!( | ||
client.release_url(), | ||
"https://api.github.com/repos/OWNER/REPO/releases/tags/SPECIFIC_TAG" | ||
); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,5 +1,6 @@ | ||
mod config; | ||
mod err; | ||
mod infra; | ||
mod model; | ||
mod sync; | ||
|
||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.